← Compose Multiplatform Component in Material Compose
DropdownMenu
Android
Desktop
A dropdown menu is a compact way of displaying multiple choices. It appears upon interaction with an element (such as an icon or button) or when users perform a specific action.
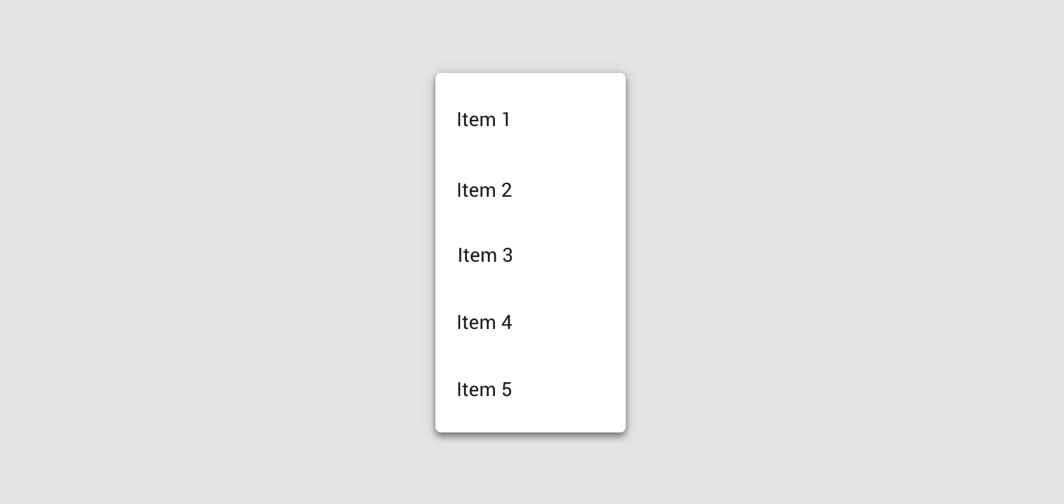
Last updated:
Installation
dependencies {
implementation("androidx.compose.material:material:1.7.0-beta04")
}
Overloads
@Composable
fun DropdownMenu(
expanded: Boolean,
onDismissRequest: () -> Unit,
modifier: Modifier = Modifier,
offset: DpOffset = DpOffset(0.dp, 0.dp),
scrollState: ScrollState = rememberScrollState(),
properties: PopupProperties = PopupProperties(focusable = true),
content: @Composable ColumnScope.() -> Unit
)
Parameters
name | description |
---|---|
expanded | whether the menu is expanded or not |
onDismissRequest | called when the user requests to dismiss the menu, such as by tapping outside the menu's bounds |
modifier | [Modifier] to be applied to the menu's content |
offset | [DpOffset] from the original position of the menu. The offset respects the [LayoutDirection], so the offset's x position will be added in LTR and subtracted in RTL. |
scrollState | a [ScrollState] to used by the menu's content for items vertical scrolling |
properties | [PopupProperties] for further customization of this popup's behavior |
content | the content of this dropdown menu, typically a [DropdownMenuItem] |
@Composable
@Deprecated(
level = DeprecationLevel.HIDDEN,
replaceWith = ReplaceWith(
expression = "DropdownMenu(expanded,onDismissRequest, focusable, modifier, offset, " +
"rememberScrollState(), content)",
"androidx.compose.foundation.rememberScrollState"
),
message = "Replaced by a DropdownMenu function with a ScrollState parameter"
fun DropdownMenu(
expanded: Boolean,
onDismissRequest: () -> Unit,
focusable: Boolean = true,
modifier: Modifier = Modifier,
offset: DpOffset = DpOffset(0.dp, 0.dp),
content: @Composable ColumnScope.() -> Unit
)
Parameters
name | description |
---|---|
expanded | Whether the menu is currently open and visible to the user |
onDismissRequest | Called when the user requests to dismiss the menu, such as by tapping outside the menu's bounds |
focusable | Whether the dropdown can capture focus |
modifier | Modifier for the menu |
offset | [DpOffset] to be added to the position of the menu |
content | content lambda |
@Composable
fun DropdownMenu(
expanded: Boolean,
onDismissRequest: () -> Unit,
focusable: Boolean = true,
modifier: Modifier = Modifier,
offset: DpOffset = DpOffset(0.dp, 0.dp),
scrollState: ScrollState = rememberScrollState(),
content: @Composable ColumnScope.() -> Unit
)
Parameters
name | description |
---|---|
expanded | Whether the menu is currently open and visible to the user |
onDismissRequest | Called when the user requests to dismiss the menu, such as by tapping outside the menu's bounds |
focusable | Whether the dropdown can capture focus |
modifier | [Modifier] to be applied to the menu's content |
offset | [DpOffset] to be added to the position of the menu |
scrollState | a [ScrollState] to used by the menu's content for items vertical scrolling |
content | the content of this dropdown menu, typically a [DropdownMenuItem] |
@Composable
@Deprecated(
level = DeprecationLevel.HIDDEN,
replaceWith = ReplaceWith(
expression = "DropdownMenu(expanded,onDismissRequest, modifier, offset, " +
"rememberScrollState(), properties, content)",
"androidx.compose.foundation.rememberScrollState"
),
message = "Replaced by a DropdownMenu function with a ScrollState parameter"
fun DropdownMenu(
expanded: Boolean,
onDismissRequest: () -> Unit,
modifier: Modifier = Modifier,
offset: DpOffset = DpOffset(0.dp, 0.dp),
properties: PopupProperties = PopupProperties(focusable = true),
content: @Composable ColumnScope.() -> Unit
)
Code Examples
MenuSample
@Composable
@Sampled
fun MenuSample() {
var expanded by remember { mutableStateOf(false) }
Box(modifier = Modifier
.fillMaxSize()
.wrapContentSize(Alignment.TopStart)) {
IconButton(onClick = { expanded = true }) {
Icon(Icons.Default.MoreVert, contentDescription = "Localized description")
}
DropdownMenu(
expanded = expanded,
onDismissRequest = { expanded = false }
) {
DropdownMenuItem(onClick = { /* Handle refresh! */ }) {
Text("Refresh")
}
DropdownMenuItem(onClick = { /* Handle settings! */ }) {
Text("Settings")
}
Divider()
DropdownMenuItem(onClick = { /* Handle send feedback! */ }) {
Text("Send Feedback")
}
}
}
}
MenuWithScrollStateSample
@Composable
@Sampled
fun MenuWithScrollStateSample() {
var expanded by remember { mutableStateOf(false) }
val scrollState = rememberScrollState()
Box(
modifier = Modifier
.fillMaxSize()
.wrapContentSize(Alignment.TopStart)
) {
IconButton(onClick = { expanded = true }) {
Icon(Icons.Default.MoreVert, contentDescription = "Localized description")
}
DropdownMenu(
expanded = expanded,
onDismissRequest = { expanded = false },
scrollState = scrollState
) {
repeat(30) {
DropdownMenuItem(onClick = { /* Handle item! */ }) {
Text("Item ${it + 1}")
}
}
}
LaunchedEffect(expanded) {
if (expanded) {
// Scroll to show the bottom menu items.
scrollState.scrollTo(scrollState.maxValue)
}
}
}
}