← Back to Material Compose
Card
Component
in
Material
. Since 0.1.0-dev15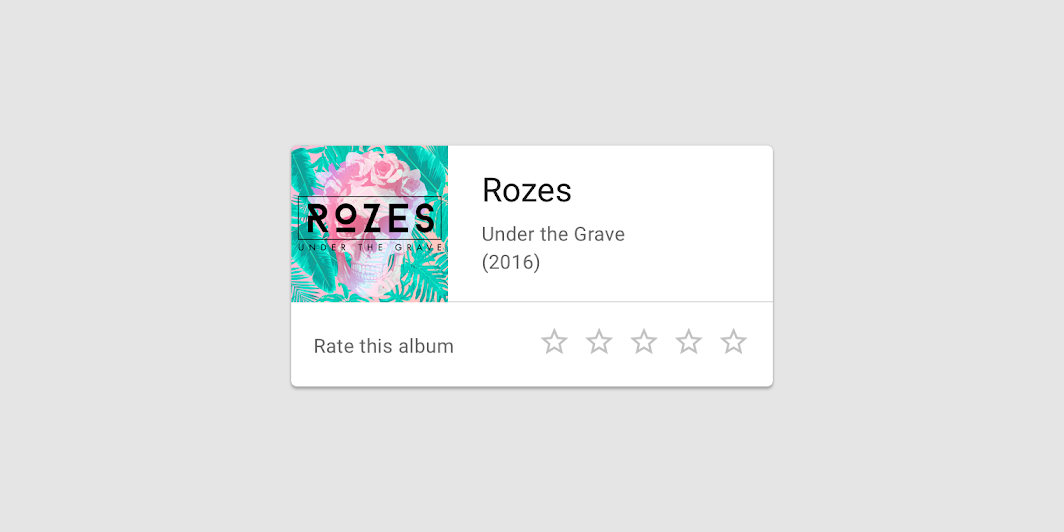
Overview
Code Examples
<a href="https://material.io/components/cards" class="external" target="_blank">Material Design card</a>.
Cards contain content and actions about a single subject.
!Cards image(https://developer.android.com/images/reference/androidx/compose/material/cards.png)
This version of Card will block clicks behind it. For clickable card, please use another overload that accepts onClick
as a parameter.
Overloads
Card
@Composable
@NonRestartableComposable
fun Card(
modifier: Modifier = Modifier,
shape: Shape = MaterialTheme.shapes.medium,
backgroundColor: Color = MaterialTheme.colors.surface,
contentColor: Color = contentColorFor(backgroundColor),
border: BorderStroke? = null,
elevation: Dp = 1.dp,
content: @Composable () -> Unit
)
Parameters
Name | Description |
---|---|
modifier | Modifier to be applied to the layout of the card. |
shape | Defines the card's shape as well its shadow. A shadow is only displayed if the elevation is greater than zero. |
backgroundColor | The background color. |
contentColor | The preferred content color provided by this card to its children. Defaults to either the matching content color for backgroundColor, or if backgroundColor is not a color from the theme, this will keep the same value set above this card. |
border | Optional border to draw on top of the card |
elevation | The z-coordinate at which to place this card. This controls the size of the shadow below the card |
Card
@ExperimentalMaterialApi
@Composable
@NonRestartableComposable
fun Card(
onClick: () -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
shape: Shape = MaterialTheme.shapes.medium,
backgroundColor: Color = MaterialTheme.colors.surface,
contentColor: Color = contentColorFor(backgroundColor),
border: BorderStroke? = null,
elevation: Dp = 1.dp,
interactionSource: MutableInteractionSource = remember { MutableInteractionSource() },
content: @Composable () -> Unit
)
Parameters
Name | Description |
---|---|
onClick | callback to be called when the card is clicked |
modifier | Modifier to be applied to the layout of the card. |
enabled | Controls the enabled state of the card. When false , this card will not be clickable |
shape | Defines the card's shape as well its shadow. A shadow is only displayed if the elevation is greater than zero. |
backgroundColor | The background color. |
contentColor | The preferred content color provided by this card to its children. Defaults to either the matching content color for backgroundColor, or if backgroundColor is not a color from the theme, this will keep the same value set above this card. |
border | Optional border to draw on top of the card |
elevation | The z-coordinate at which to place this card. This controls the size of the shadow below the card. |
interactionSource | the MutableInteractionSource representing the stream of Interactions for this Card. You can create and pass in your own remembered MutableInteractionSource if you want to observe Interactions and customize the appearance / behavior of this card in different Interactions |