← Compose Multiplatform Component in Material 3 Compose
DropdownMenuItem
Common
Android
Menus display a list of choices on a temporary surface. They appear when users interact with a button, action, or other control.
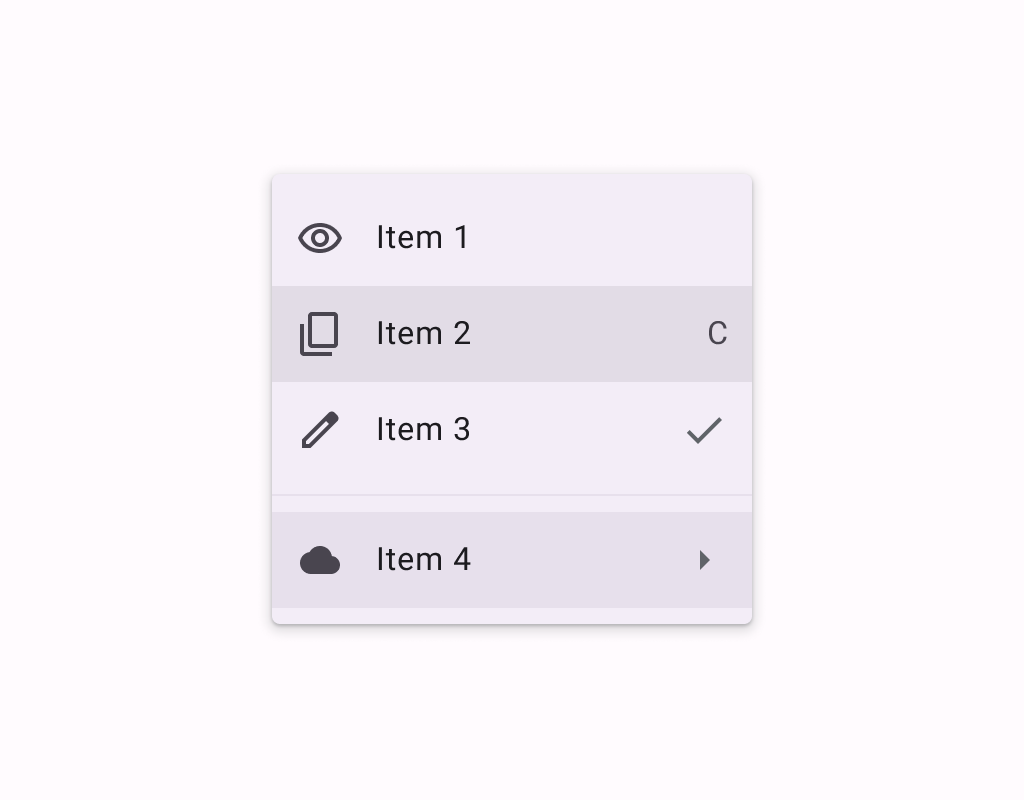
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.3.0-beta04")
}
Overloads
@Composable
fun DropdownMenuItem(
text: @Composable () -> Unit,
onClick: () -> Unit,
modifier: Modifier = Modifier,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
enabled: Boolean = true,
colors: MenuItemColors = MenuDefaults.itemColors(),
contentPadding: PaddingValues = MenuDefaults.DropdownMenuItemContentPadding,
interactionSource: MutableInteractionSource? = null,
)
Parameters
name | description |
---|---|
text | text of the menu item |
onClick | called when this menu item is clicked |
modifier | the [Modifier] to be applied to this menu item |
leadingIcon | optional leading icon to be displayed at the beginning of the item's text |
trailingIcon | optional trailing icon to be displayed at the end of the item's text. This trailing icon slot can also accept [Text] to indicate a keyboard shortcut. |
enabled | controls the enabled state of this menu item. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
colors | [MenuItemColors] that will be used to resolve the colors used for this menu item in different states. See [MenuDefaults.itemColors]. |
contentPadding | the padding applied to the content of this menu item |
interactionSource | an optional hoisted [MutableInteractionSource] for observing and emitting [Interaction]s for this menu item. You can use this to change the menu item's appearance or preview the menu item in different states. Note that if null is provided, interactions will still happen internally. |
@Composable
fun DropdownMenuItem(
text: @Composable () -> Unit,
onClick: () -> Unit,
modifier: Modifier,
leadingIcon: @Composable (() -> Unit)?,
trailingIcon: @Composable (() -> Unit)?,
enabled: Boolean,
colors: MenuItemColors,
contentPadding: PaddingValues,
interactionSource: MutableInteractionSource?,
)
@Composable
fun DropdownMenuItem(
text: @Composable () -> Unit,
onClick: () -> Unit,
modifier: Modifier,
leadingIcon: @Composable (() -> Unit)?,
trailingIcon: @Composable (() -> Unit)?,
enabled: Boolean,
colors: MenuItemColors,
contentPadding: PaddingValues,
interactionSource: MutableInteractionSource?,
)
Code Example
MenuSample
@Composable
@Sampled
@Preview
fun MenuSample() {
var expanded by remember { mutableStateOf(false) }
Box(modifier = Modifier.fillMaxSize().wrapContentSize(Alignment.TopStart)) {
IconButton(onClick = { expanded = true }) {
Icon(Icons.Default.MoreVert, contentDescription = "Localized description")
}
DropdownMenu(expanded = expanded, onDismissRequest = { expanded = false }) {
DropdownMenuItem(
text = { Text("Edit") },
onClick = { /* Handle edit! */ },
leadingIcon = { Icon(Icons.Outlined.Edit, contentDescription = null) }
)
DropdownMenuItem(
text = { Text("Settings") },
onClick = { /* Handle settings! */ },
leadingIcon = { Icon(Icons.Outlined.Settings, contentDescription = null) }
)
HorizontalDivider()
DropdownMenuItem(
text = { Text("Send Feedback") },
onClick = { /* Handle send feedback! */ },
leadingIcon = { Icon(Icons.Outlined.Email, contentDescription = null) },
trailingIcon = { Text("F11", textAlign = TextAlign.Center) }
)
}
}
}