← Compose Multiplatform Component in Material 3 Compose
ElevatedAssistChip
Common
Chips help people enter information, make selections, filter content, or trigger actions. Chips can show multiple interactive elements together in the same area, such as a list of selectable movie times, or a series of email contacts.
Assist chips represent smart or automated actions that can span multiple apps, such as opening a calendar event from the home screen. Assist chips function as though the user asked an assistant to complete the action. They should appear dynamically and contextually in a UI.
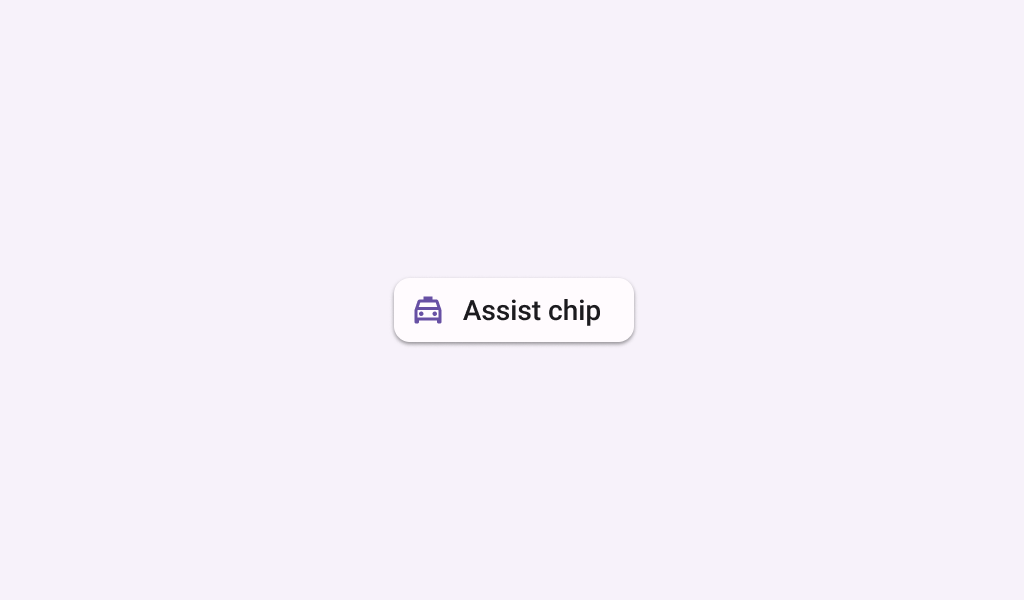
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.3.0-beta04")
}
Overloads
@Composable
fun ElevatedAssistChip(
onClick: () -> Unit,
label: @Composable () -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
shape: Shape = AssistChipDefaults.shape,
colors: ChipColors = AssistChipDefaults.elevatedAssistChipColors(),
elevation: ChipElevation? = AssistChipDefaults.elevatedAssistChipElevation(),
border: BorderStroke? = null,
interactionSource: MutableInteractionSource? = null
)
Parameters
name | description |
---|---|
onClick | called when this chip is clicked |
label | text label for this chip |
modifier | the [Modifier] to be applied to this chip |
enabled | controls the enabled state of this chip. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
leadingIcon | optional icon at the start of the chip, preceding the [label] text |
trailingIcon | optional icon at the end of the chip |
shape | defines the shape of this chip's container, border (when [border] is not null), and shadow (when using [elevation]) |
colors | [ChipColors] that will be used to resolve the colors used for this chip in different states. See [AssistChipDefaults.elevatedAssistChipColors]. |
elevation | [ChipElevation] used to resolve the elevation for this chip in different states. This controls the size of the shadow below the chip. Additionally, when the container color is [ColorScheme.surface], this controls the amount of primary color applied as an overlay. See [AssistChipDefaults.elevatedAssistChipElevation]. |
border | the border to draw around the container of this chip |
interactionSource | an optional hoisted [MutableInteractionSource] for observing and emitting [Interaction]s for this chip. You can use this to change the chip's appearance or preview the chip in different states. Note that if null is provided, interactions will still happen internally. |
@Composable
@Deprecated(
"Maintained for binary compatibility. Use version with ElevatedAssistChip that take a" +
" BorderStroke instead",
replaceWith =
ReplaceWith(
"ElevatedAssistChip(onClick, label, modifier, enabled," +
"leadingIcon, trailingIcon, shape, colors, elevation, border, interactionSource"
),
level = DeprecationLevel.HIDDEN
@Suppress("DEPRECATION"
fun ElevatedAssistChip(
onClick: () -> Unit,
label: @Composable () -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
shape: Shape = AssistChipDefaults.shape,
colors: ChipColors = AssistChipDefaults.elevatedAssistChipColors(),
elevation: ChipElevation? = AssistChipDefaults.elevatedAssistChipElevation(),
border: ChipBorder? = null,
interactionSource: MutableInteractionSource = remember { MutableInteractionSource() }
)
Parameters
name | description |
---|---|
onClick | called when this chip is clicked |
label | text label for this chip |
modifier | the [Modifier] to be applied to this chip |
enabled | controls the enabled state of this chip. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
leadingIcon | optional icon at the start of the chip, preceding the [label] text |
trailingIcon | optional icon at the end of the chip |
shape | defines the shape of this chip's container, border (when [border] is not null), and shadow (when using [elevation]) |
colors | [ChipColors] that will be used to resolve the colors used for this chip in different states. See [AssistChipDefaults.elevatedAssistChipColors]. |
elevation | [ChipElevation] used to resolve the elevation for this chip in different states. This controls the size of the shadow below the chip. Additionally, when the container color is [ColorScheme.surface], this controls the amount of primary color applied as an overlay. See [AssistChipDefaults.elevatedAssistChipElevation]. |
border | the border to draw around the container of this chip |
interactionSource | the [MutableInteractionSource] representing the stream of [Interaction]s for this chip. You can create and pass in your own remember ed instance to observe [Interaction]s and customize the appearance / behavior of this chip in different states. |
Code Example
ElevatedAssistChipSample
@Composable
@Sampled
@Preview
fun ElevatedAssistChipSample() {
ElevatedAssistChip(
onClick = { /* Do something! */ },
label = { Text("Assist Chip") },
leadingIcon = {
Icon(
Icons.Filled.Settings,
contentDescription = "Localized description",
Modifier.size(AssistChipDefaults.IconSize)
)
}
)
}