← Compose Multiplatform Component in Material 3 Compose
LinearProgressIndicator
Common
Progress indicators express an unspecified wait time or display the duration of a process.
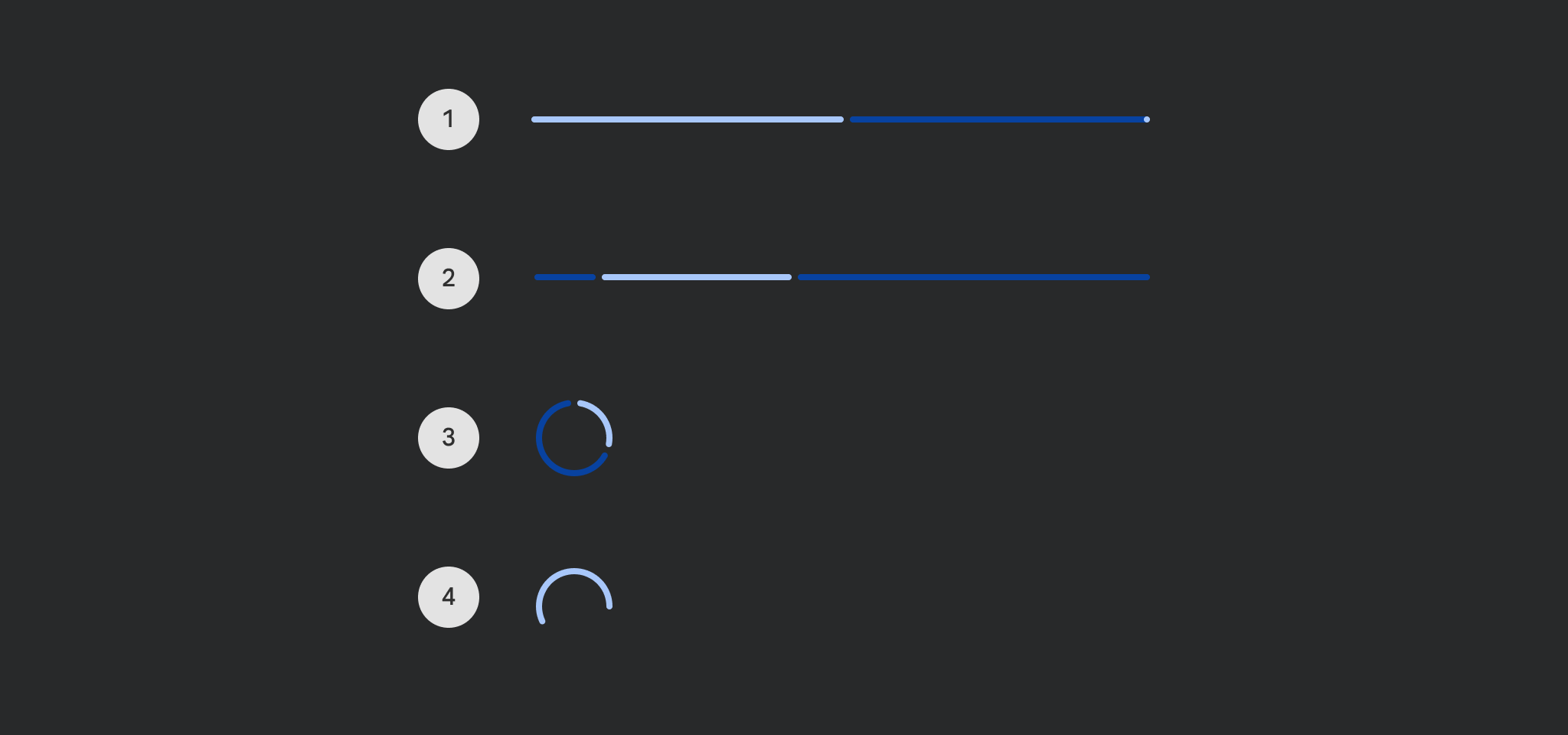
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.3.0-beta04")
}
Overloads
@Composable
@OptIn(ExperimentalMaterial3Api::class
@Deprecated(
message =
"Use the overload that takes `gapSize` and `drawStopIndicator`, see " +
"`LegacyLinearProgressIndicatorSample` on how to restore the previous behavior",
replaceWith =
ReplaceWith(
"LinearProgressIndicator(progress, modifier, color, trackColor, strokeCap, " +
"gapSize, drawStopIndicator)"
),
level = DeprecationLevel.HIDDEN
fun LinearProgressIndicator(
progress: () -> Float,
modifier: Modifier = Modifier,
color: Color = ProgressIndicatorDefaults.linearColor,
trackColor: Color = ProgressIndicatorDefaults.linearTrackColor,
strokeCap: StrokeCap = ProgressIndicatorDefaults.LinearStrokeCap,
)
Parameters
name | description |
---|---|
progress | the progress of this progress indicator, where 0.0 represents no progress and 1.0 represents full progress. Values outside of this range are coerced into the range. |
modifier | the [Modifier] to be applied to this progress indicator |
color | color of this progress indicator |
trackColor | color of the track behind the indicator, visible when the progress has not reached the area of the overall indicator yet |
strokeCap | stroke cap to use for the ends of this progress indicator |
@Composable
@OptIn(ExperimentalMaterial3Api::class
fun LinearProgressIndicator(
progress: () -> Float,
modifier: Modifier = Modifier,
color: Color = ProgressIndicatorDefaults.linearColor,
trackColor: Color = ProgressIndicatorDefaults.linearTrackColor,
strokeCap: StrokeCap = ProgressIndicatorDefaults.LinearStrokeCap,
gapSize: Dp = ProgressIndicatorDefaults.LinearIndicatorTrackGapSize,
drawStopIndicator: DrawScope.() -> Unit = {
drawStopIndicator(
drawScope = this,
stopSize = ProgressIndicatorDefaults.LinearTrackStopIndicatorSize,
color = color,
strokeCap = strokeCap
)
},
)
Parameters
name | description |
---|---|
progress | the progress of this progress indicator, where 0.0 represents no progress and 1.0 represents full progress. Values outside of this range are coerced into the range. |
modifier | the [Modifier] to be applied to this progress indicator |
color | color of this progress indicator |
trackColor | color of the track behind the indicator, visible when the progress has not reached the area of the overall indicator yet |
strokeCap | stroke cap to use for the ends of this progress indicator |
gapSize | size of the gap between the progress indicator and the track |
drawStopIndicator | lambda that will be called to draw the stop indicator |
@Composable
@OptIn(ExperimentalMaterial3Api::class
@Deprecated(
message =
"Use the overload that takes `gapSize`, see `" +
"LegacyIndeterminateLinearProgressIndicatorSample` on how to restore the previous behavior",
replaceWith =
ReplaceWith("LinearProgressIndicator(modifier, color, trackColor, strokeCap, gapSize)"),
level = DeprecationLevel.HIDDEN
fun LinearProgressIndicator(
modifier: Modifier = Modifier,
color: Color = ProgressIndicatorDefaults.linearColor,
trackColor: Color = ProgressIndicatorDefaults.linearTrackColor,
strokeCap: StrokeCap = ProgressIndicatorDefaults.LinearStrokeCap,
)
Parameters
name | description |
---|---|
modifier | the [Modifier] to be applied to this progress indicator |
color | color of this progress indicator |
trackColor | color of the track behind the indicator, visible when the progress has not reached the area of the overall indicator yet |
strokeCap | stroke cap to use for the ends of this progress indicator |
@Composable
@OptIn(ExperimentalMaterial3Api::class
fun LinearProgressIndicator(
modifier: Modifier = Modifier,
color: Color = ProgressIndicatorDefaults.linearColor,
trackColor: Color = ProgressIndicatorDefaults.linearTrackColor,
strokeCap: StrokeCap = ProgressIndicatorDefaults.LinearStrokeCap,
gapSize: Dp = ProgressIndicatorDefaults.LinearIndicatorTrackGapSize,
)
Parameters
name | description |
---|---|
modifier | the [Modifier] to be applied to this progress indicator |
color | color of this progress indicator |
trackColor | color of the track behind the indicator, visible when the progress has not reached the area of the overall indicator yet |
strokeCap | stroke cap to use for the ends of this progress indicator |
gapSize | size of the gap between the progress indicator and the track |
@Composable
@Deprecated("Maintained for binary compatibility", level = DeprecationLevel.HIDDEN
fun LinearProgressIndicator(
modifier: Modifier = Modifier,
color: Color = ProgressIndicatorDefaults.linearColor,
trackColor: Color = ProgressIndicatorDefaults.linearTrackColor,
)
@Composable
@Deprecated("Maintained for binary compatibility", level = DeprecationLevel.HIDDEN
@Suppress("DEPRECATION"
fun LinearProgressIndicator(
progress: Float,
modifier: Modifier = Modifier,
color: Color = ProgressIndicatorDefaults.linearColor,
trackColor: Color = ProgressIndicatorDefaults.linearTrackColor,
)
@Composable
@Deprecated(
message = "Use the overload that takes `progress` as a lambda",
replaceWith =
ReplaceWith(
"LinearProgressIndicator(\n" +
"progress = { progress },\n" +
"modifier = modifier,\n" +
"color = color,\n" +
"trackColor = trackColor,\n" +
"strokeCap = strokeCap,\n" +
")"
)
fun LinearProgressIndicator(
progress: Float,
modifier: Modifier = Modifier,
color: Color = ProgressIndicatorDefaults.linearColor,
trackColor: Color = ProgressIndicatorDefaults.linearTrackColor,
strokeCap: StrokeCap = ProgressIndicatorDefaults.LinearStrokeCap,
)
Code Examples
LinearProgressIndicatorSample
@Composable
@Sampled
@Preview
fun LinearProgressIndicatorSample() {
var progress by remember { mutableStateOf(0.1f) }
val animatedProgress by
animateFloatAsState(
targetValue = progress,
animationSpec = ProgressIndicatorDefaults.ProgressAnimationSpec
)
Column(horizontalAlignment = Alignment.CenterHorizontally) {
LinearProgressIndicator(
progress = { animatedProgress },
)
Spacer(Modifier.requiredHeight(30.dp))
Text("Set progress:")
Slider(
modifier = Modifier.width(300.dp),
value = progress,
valueRange = 0f..1f,
onValueChange = { progress = it },
)
}
}
IndeterminateLinearProgressIndicatorSample
@Composable
@Sampled
@Preview
fun IndeterminateLinearProgressIndicatorSample() {
Column(horizontalAlignment = Alignment.CenterHorizontally) { LinearProgressIndicator() }
}