← Back to Material 3 Compose
ModalNavigationDrawer
Component
in
Material 3
. Since 1.0.0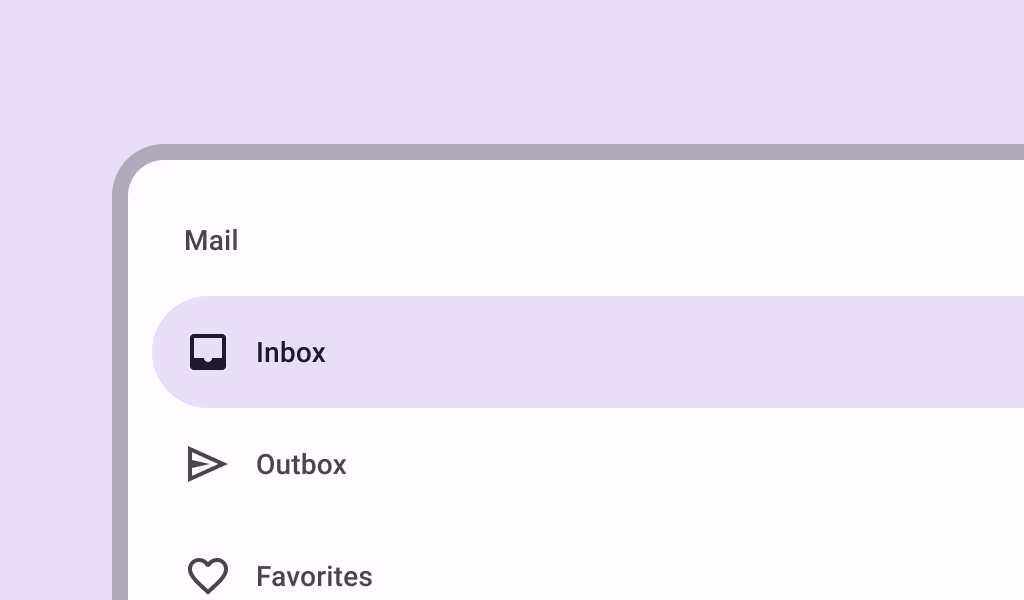
Overview
Code Examples
Video
<a href="https://m3.material.io/components/navigation-drawer/overview" class="external" target="_blank">Material Design navigation drawer</a>.
Navigation drawers provide ergonomic access to destinations in an app.
Modal navigation drawers block interaction with the rest of an app’s content with a scrim. They are elevated above most of the app’s UI and don’t affect the screen’s layout grid.
!Navigation drawer image(https://developer.android.com/images/reference/androidx/compose/material3/navigation-drawer.png)
Overloads
ModalNavigationDrawer
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun ModalNavigationDrawer(
drawerContent: @Composable () -> Unit,
modifier: Modifier = Modifier,
drawerState: DrawerState = rememberDrawerState(DrawerValue.Closed),
gesturesEnabled: Boolean = true,
scrimColor: Color = DrawerDefaults.scrimColor,
content: @Composable () -> Unit
)
Parameters
Name | Description |
---|---|
drawerContent | content inside this drawer |
modifier | the Modifier to be applied to this drawer |
drawerState | state of the drawer |
gesturesEnabled | whether or not the drawer can be interacted by gestures |
scrimColor | color of the scrim that obscures content when the drawer is open |
content | content of the rest of the U |