← Back to Material 3 Compose
OutlinedTextField
Component
in
Material 3
. Since 1.0.0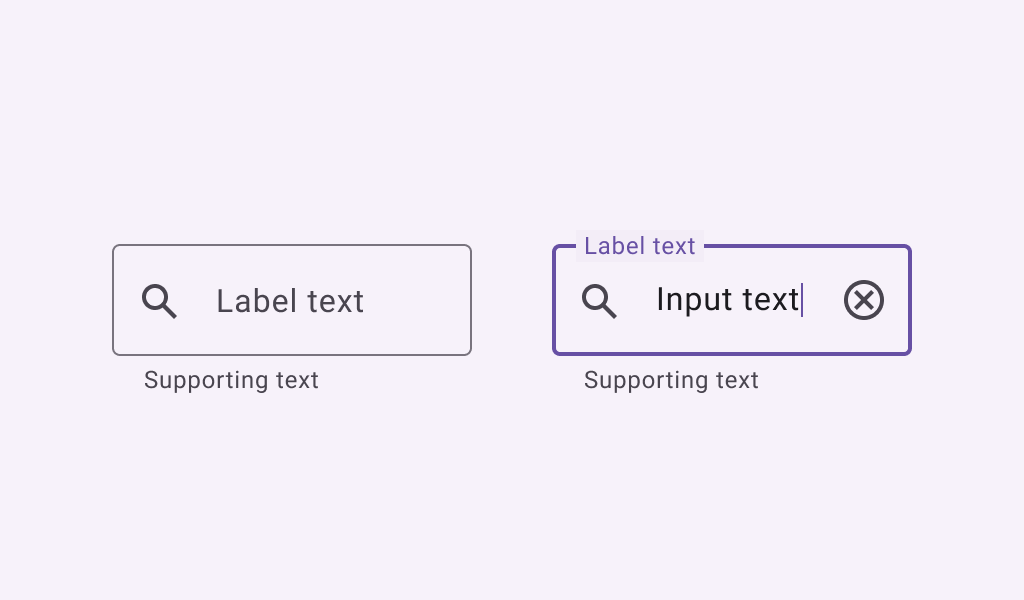
Overview
Code Examples
Video
<a href="https://m3.material.io/components/text-fields/overview" class="external" target="_blank">Material Design outlined text field</a>.
Text fields allow users to enter text into a UI. They typically appear in forms and dialogs. Outlined text fields have less visual emphasis than filled text fields. When they appear in places like forms, where many text fields are placed together, their reduced emphasis helps simplify the layout.
!Outlined text field image(https://developer.android.com/images/reference/androidx/compose/material3/outlined-text-field.png)
See example usage:
Overloads
OutlinedTextField
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun OutlinedTextField(
value: String,
onValueChange: (String) -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
readOnly: Boolean = false,
textStyle: TextStyle = LocalTextStyle.current,
label: @Composable (() -> Unit)? = null,
placeholder: @Composable (() -> Unit)? = null,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
prefix: @Composable (() -> Unit)? = null,
suffix: @Composable (() -> Unit)? = null,
supportingText: @Composable (() -> Unit)? = null,
isError: Boolean = false,
visualTransformation: VisualTransformation = VisualTransformation.None,
keyboardOptions: KeyboardOptions = KeyboardOptions.Default,
keyboardActions: KeyboardActions = KeyboardActions.Default,
singleLine: Boolean = false,
maxLines: Int = if (singleLine) 1 else Int.MAX_VALUE,
minLines: Int = 1,
interactionSource: MutableInteractionSource = remember { MutableInteractionSource() },
shape: Shape = OutlinedTextFieldDefaults.shape,
colors: TextFieldColors = OutlinedTextFieldDefaults.colors()
)
Parameters
Name | Description |
---|---|
value | the input text to be shown in the text field |
onValueChange | the callback that is triggered when the input service updates the text. An updated text comes as a parameter of the callback |
modifier | the Modifier to be applied to this text field |
enabled | controls the enabled state of this text field. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
readOnly | controls the editable state of the text field. When true , the text field cannot be modified. However, a user can focus it and copy text from it. Read-only text fields are usually used to display pre-filled forms that a user cannot edit. |
textStyle | the style to be applied to the input text. Defaults to LocalTextStyle. |
label | the optional label to be displayed inside the text field container. The default text style for internal Text is Typography.bodySmall when the text field is in focus and Typography.bodyLarge when the text field is not in focus |
placeholder | the optional placeholder to be displayed when the text field is in focus and the input text is empty. The default text style for internal Text is Typography.bodyLarge |
leadingIcon | the optional leading icon to be displayed at the beginning of the text field container |
trailingIcon | the optional trailing icon to be displayed at the end of the text field container |
prefix | the optional prefix to be displayed before the input text in the text field |
suffix | the optional suffix to be displayed after the input text in the text field |
supportingText | the optional supporting text to be displayed below the text field |
isError | indicates if the text field's current value is in error. If set to true, the label, bottom indicator and trailing icon by default will be displayed in error color |
visualTransformation | transforms the visual representation of the input value For example, you can use PasswordVisualTransformationandroidx.compose.ui.text.input.PasswordVisualTransformation to create a password text field. By default, no visual transformation is applied. |
keyboardOptions | software keyboard options that contains configuration such as KeyboardType and ImeAction |
keyboardActions | when the input service emits an IME action, the corresponding callback is called. Note that this IME action may be different from what you specified in KeyboardOptions.imeAction |
singleLine | when true , this text field becomes a single horizontally scrolling text field instead of wrapping onto multiple lines. The keyboard will be informed to not show the return key as the ImeAction. Note that maxLines parameter will be ignored as the maxLines attribute will be automatically set to 1. |
maxLines | the maximum height in terms of maximum number of visible lines. It is required that 1 <= minLines <= maxLines. This parameter is ignored when singleLine is true. |
minLines | the minimum height in terms of minimum number of visible lines. It is required that 1 <= minLines <= maxLines. This parameter is ignored when singleLine is true. |
interactionSource | the MutableInteractionSource representing the stream of Interactions for this text field. You can create and pass in your own remember ed instance to observe Interactions and customize the appearance / behavior of this text field in different states. |
shape | defines the shape of this text field's border |
colors | TextFieldColors that will be used to resolve the colors used for this text field in different states. See OutlinedTextFieldDefaults.colors |
OutlinedTextField
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun OutlinedTextField(
value: TextFieldValue,
onValueChange: (TextFieldValue) -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
readOnly: Boolean = false,
textStyle: TextStyle = LocalTextStyle.current,
label: @Composable (() -> Unit)? = null,
placeholder: @Composable (() -> Unit)? = null,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
prefix: @Composable (() -> Unit)? = null,
suffix: @Composable (() -> Unit)? = null,
supportingText: @Composable (() -> Unit)? = null,
isError: Boolean = false,
visualTransformation: VisualTransformation = VisualTransformation.None,
keyboardOptions: KeyboardOptions = KeyboardOptions.Default,
keyboardActions: KeyboardActions = KeyboardActions.Default,
singleLine: Boolean = false,
maxLines: Int = if (singleLine) 1 else Int.MAX_VALUE,
minLines: Int = 1,
interactionSource: MutableInteractionSource = remember { MutableInteractionSource() },
shape: Shape = OutlinedTextFieldDefaults.shape,
colors: TextFieldColors = OutlinedTextFieldDefaults.colors()
)
Parameters
Name | Description |
---|---|
value | the input TextFieldValue to be shown in the text field |
onValueChange | the callback that is triggered when the input service updates values in TextFieldValue. An updated TextFieldValue comes as a parameter of the callback |
modifier | the Modifier to be applied to this text field |
enabled | controls the enabled state of this text field. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
readOnly | controls the editable state of the text field. When true , the text field cannot be modified. However, a user can focus it and copy text from it. Read-only text fields are usually used to display pre-filled forms that a user cannot edit. |
textStyle | the style to be applied to the input text. Defaults to LocalTextStyle. |
label | the optional label to be displayed inside the text field container. The default text style for internal Text is Typography.bodySmall when the text field is in focus and Typography.bodyLarge when the text field is not in focus |
placeholder | the optional placeholder to be displayed when the text field is in focus and the input text is empty. The default text style for internal Text is Typography.bodyLarge |
leadingIcon | the optional leading icon to be displayed at the beginning of the text field container |
trailingIcon | the optional trailing icon to be displayed at the end of the text field container |
prefix | the optional prefix to be displayed before the input text in the text field |
suffix | the optional suffix to be displayed after the input text in the text field |
supportingText | the optional supporting text to be displayed below the text field |
isError | indicates if the text field's current value is in error state. If set to true, the label, bottom indicator and trailing icon by default will be displayed in error color |
visualTransformation | transforms the visual representation of the input value For example, you can use PasswordVisualTransformationandroidx.compose.ui.text.input.PasswordVisualTransformation to create a password text field. By default, no visual transformation is applied. |
keyboardOptions | software keyboard options that contains configuration such as KeyboardType and ImeAction |
keyboardActions | when the input service emits an IME action, the corresponding callback is called. Note that this IME action may be different from what you specified in KeyboardOptions.imeAction |
singleLine | when true , this text field becomes a single horizontally scrolling text field instead of wrapping onto multiple lines. The keyboard will be informed to not show the return key as the ImeAction. Note that maxLines parameter will be ignored as the maxLines attribute will be automatically set to 1. |
maxLines | the maximum height in terms of maximum number of visible lines. It is required that 1 <= minLines <= maxLines. This parameter is ignored when singleLine is true. |
minLines | the minimum height in terms of minimum number of visible lines. It is required that 1 <= minLines <= maxLines. This parameter is ignored when singleLine is true. |
interactionSource | the MutableInteractionSource representing the stream of Interactions for this text field. You can create and pass in your own remember ed instance to observe Interactions and customize the appearance / behavior of this text field in different states. |
shape | defines the shape of this text field's border |
colors | TextFieldColors that will be used to resolve the colors used for this text field in different states. See OutlinedTextFieldDefaults.colors |